How to Handle Exceptions in jBPM
Error events allow us to perform certain actions if we encounter an exception during our business process.
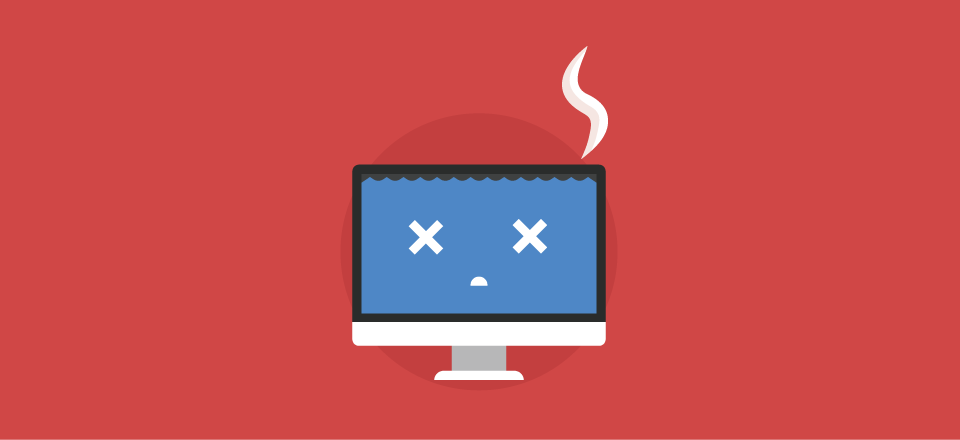
My current client is credit card company called Global Bank.
And whenever someone reports fraud, they give the customer a new account and copy over the non-fraudulent transactions.
But sometimes this process go wrong.
And if we encounter a problem during our business process, we’ll need a way to change course.
So what do we do?
Use Error Events
Error events allow us to perform certain actions if we encounter an exception during our business process. These events are built into the process so that we can visually see how errors are handled.
How to Handle Exceptions in jBPM
What You’ll Need
- JBoss Developer Studio w/ JBoss EAP 7.1+ Download here
- JBoss Maven Repositories (Section 1: Steps 4-7)
- Red Hat Process and Rules Development Stack (here)
- Basic Maven & BPM knowledge
We will create a business process that attempts to retrieve a credit card account. When that retrieval fails, our process will catch the error and perform some activity.
Review the Business Process
1: Download and import simple-error-process-starter
2: Review the business process
- This process attempts to retrieve an account from an external service
3: Review AccountWorkItemHandler
- This retrieves an account from the database. But if we can’t find the account number, we throw an exception
Create an Error Handling Sub-Process
Step 1: Add the SignalingTaskHandlerDecorator to the kie-deployment description
- This built in class wraps around any work item handler and automatically signals an error event when we throw an Exception
- The constructor takes in a work item handler and an error code. We’ll use this code when catch the error in our process
- Replace line 6 with:
<identifier>new org.jbpm.bpmn2.handler.SignallingTaskHandlerDecorator(new org.codelikethewind.workitemhandler.AccountWorkItemHandler(), "Error-accountError")</identifier>
- The error code format is Error-<your_error_code>
Step 2: Define the error in our business process
- Open the business process
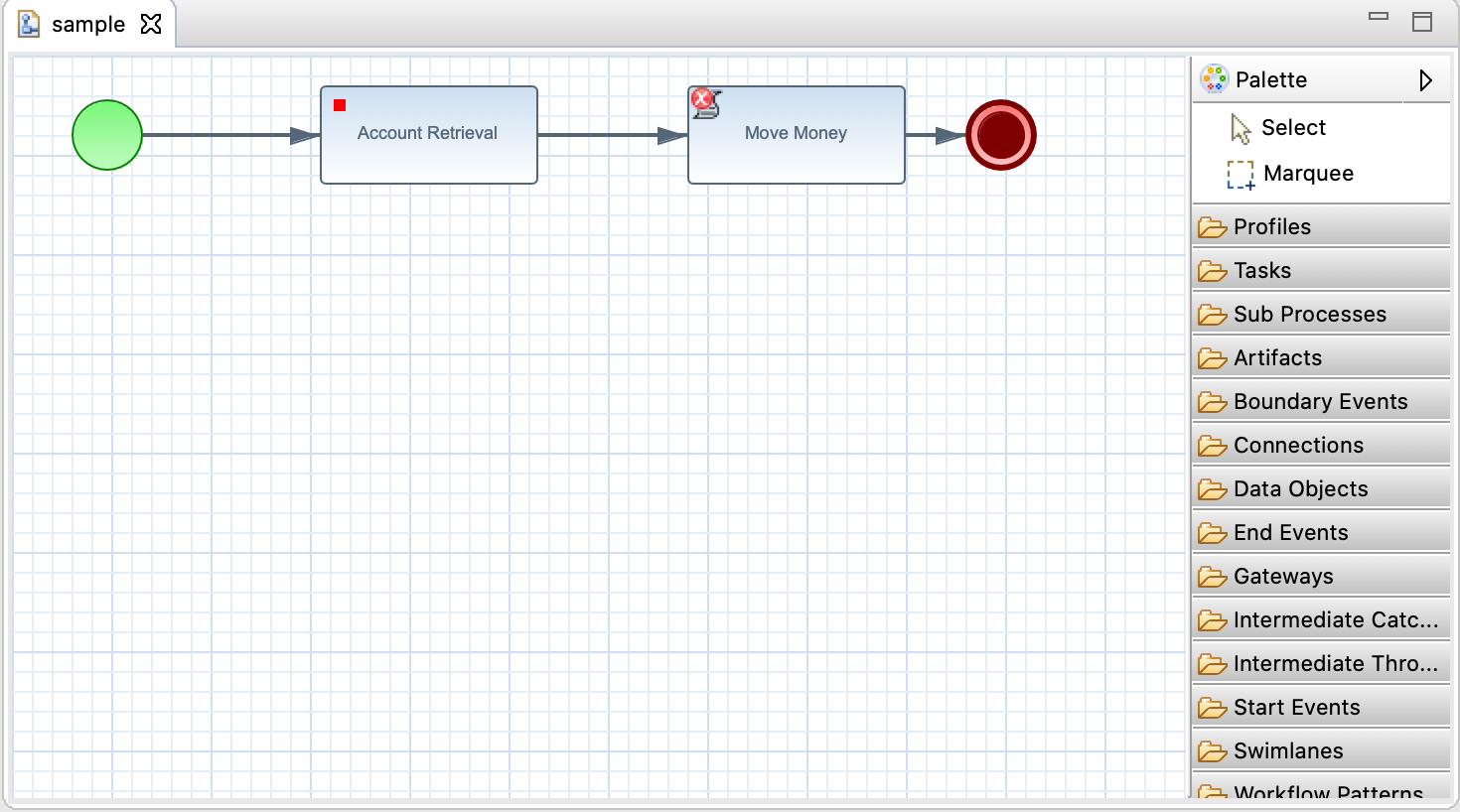
- Click anywhere in the process → Properties → Definitions
- Add a new Error called “error”. Give it our code: accountError
- Add a new data type: org.kie.api.runtime.process.WorkItem
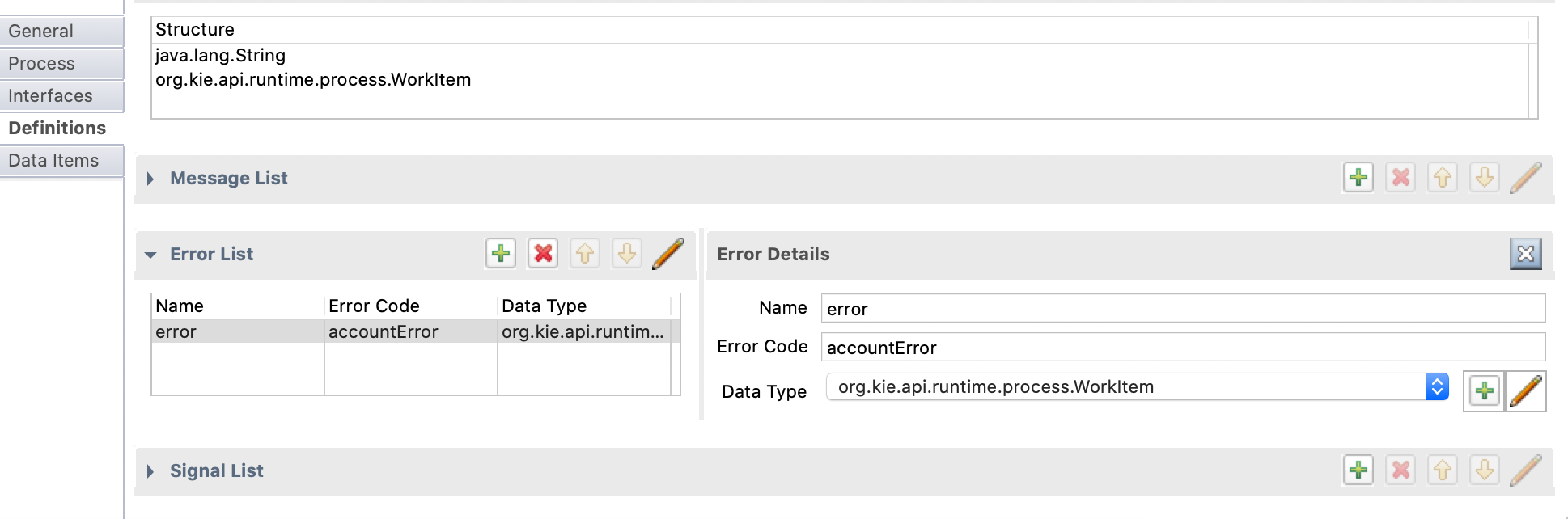
Step 3: Add a variable to store the exception
- Click Data Items
- Under Local Variables, add a variable called exceptionWorkItem
- Use the data type: org.kie.api.runtime.process.WorkItem
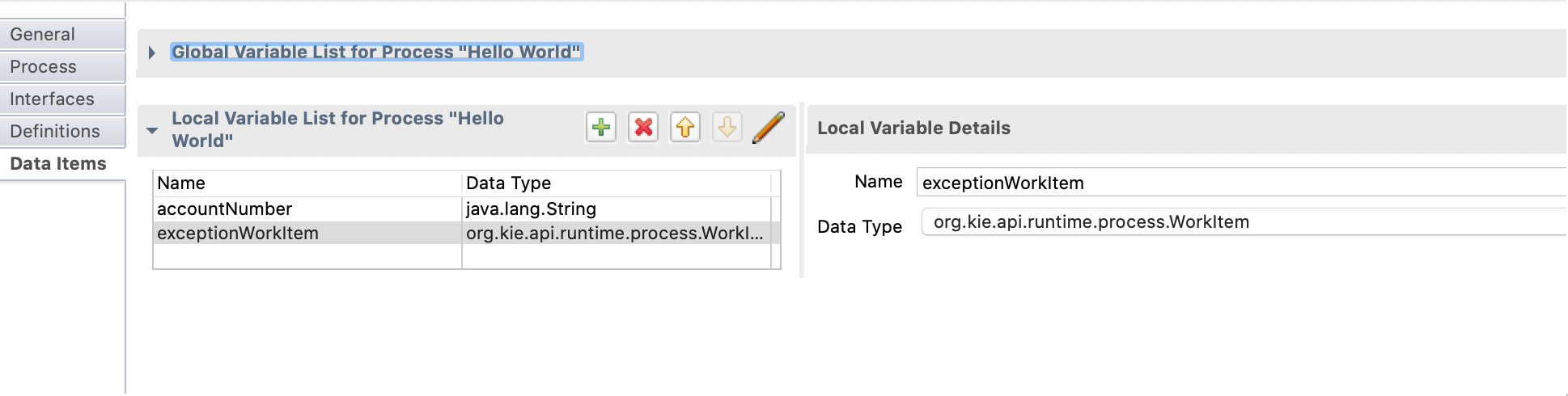
Step 4: Catch the error and handle it
From the palette, drag an Event Sub-Process to your process
- Event sub processes are triggered by an event. In our case, that’s an error event
Drag an Error start event to your sub-process
- Double click the error event
- Double click the event type and select the error we created
- Change the target to our exception variable
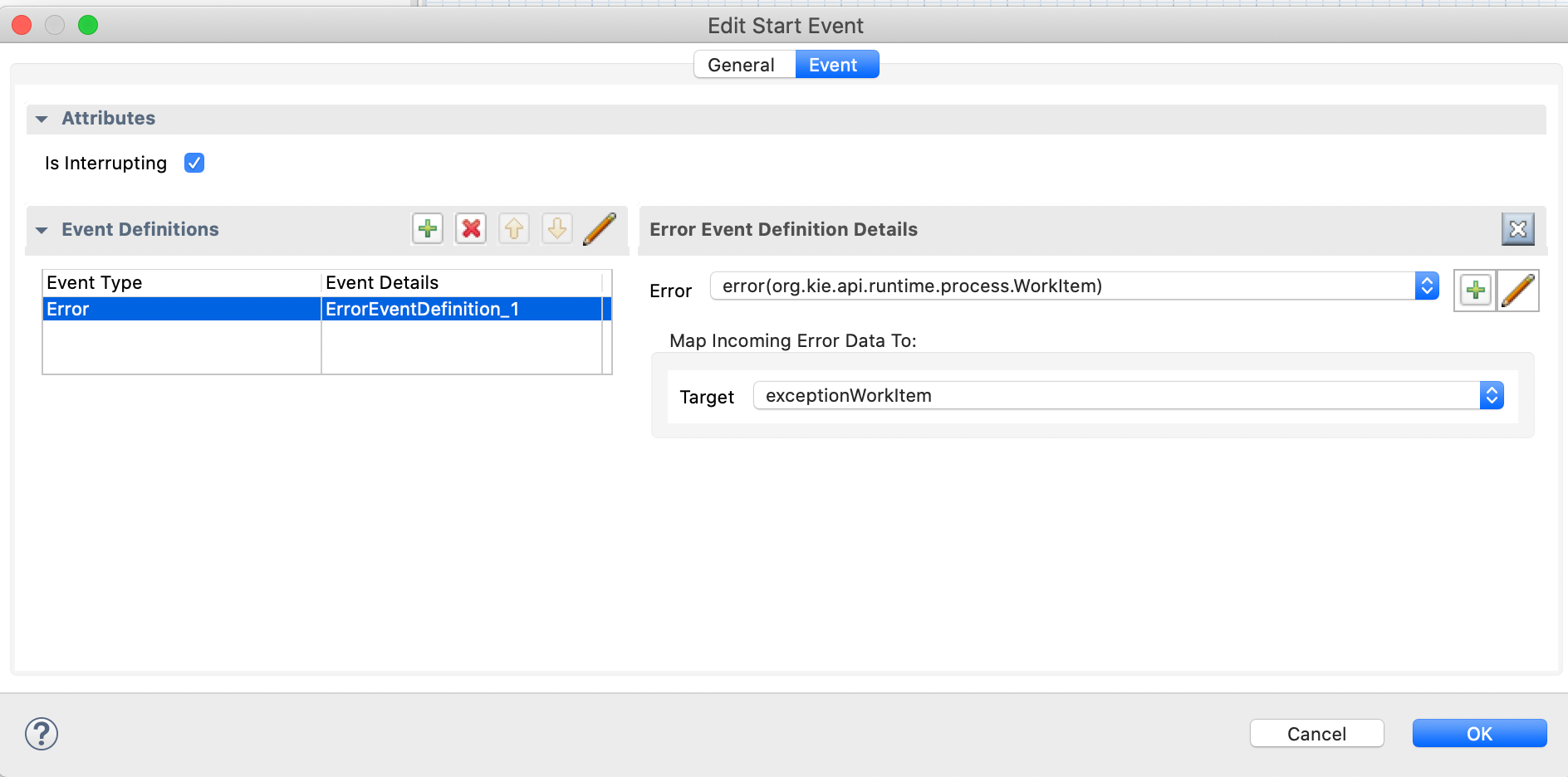
From the custom tasks, drag a “Error Handling” task to the sub-process
- This custom task logs the exception. You can execute anything with this though.
- Double click the custom task and go to I/O Parameters
- Add the exceptionWorkItem as a parameter
- Connect the error event to the Error handling task
- Connect a terminate end event to the Error handling task
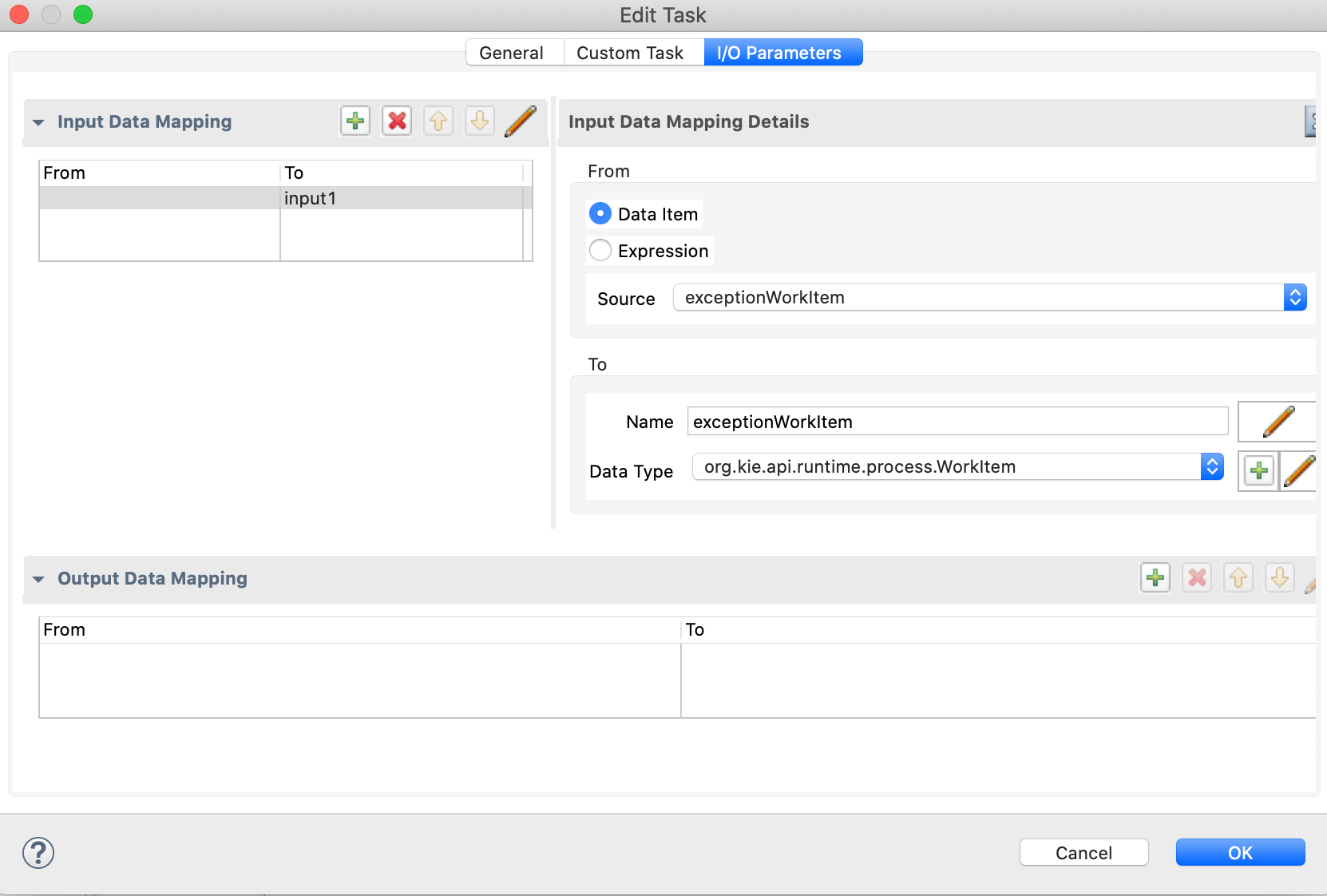
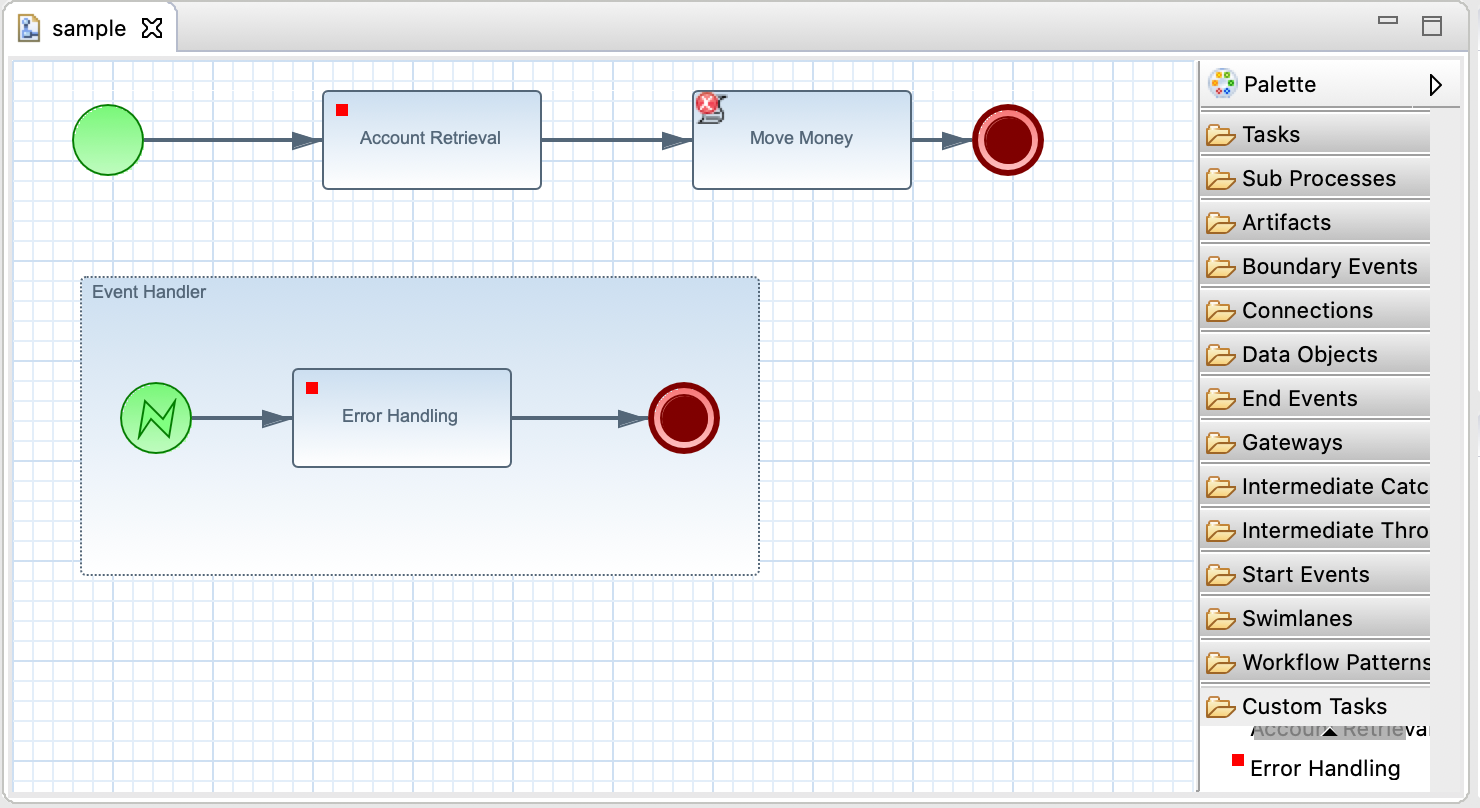
Step 5: Save the process and build
- Run mvn clean install
Run the Error-Handling Process
Step 1: Deploy the simple-embedded-process to your server
- Download the project & run mvn clean install
- This project runs a deployed process
- Deploy using Method 1 here. If you’re on EAP 7, be sure to start your server with the full profile
Step 2: Run the process
- Go to http://localhost:8080/simple-embedded-process
- Click “Start Process”
Step 3: Verify our exception logic ran
- Check the logs. You should see “Handling exception caused by work item..” followed by a logged exception
Recap
Exception handling helps us perform corrective (or informative) action when something goes wrong in our process. SignalingTaskHandlerDecorator and Error events are a few built in tools to make throwing and catching exceptions easy.
Source Code & Links
Happy Coding!
-T.O.