How to Embed a jBPM Process in a Java EE Application
jBPM APIs allow you to manage complex processes without a dedicated server
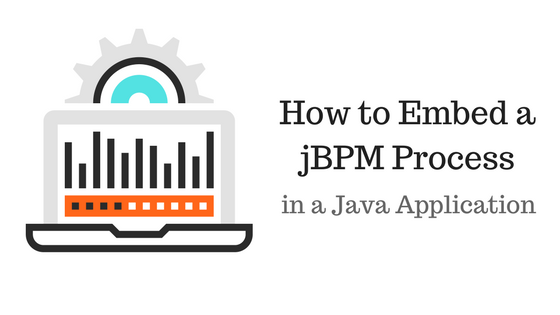
You have some nasty business workflows that need to be tamed.
And you've heard how easy it is to build, visualize, and modify complex processes with JBoss BPMS (now called Process Automation Manager).
But even though you want this functionality, you don’t have the resources to set up a standalone server.
So..what do you do?
BPMS, Without a Dedicated Server
If you want process management without a standalone server, then you can..
Embed JBPM in Your Application
You can use the JBPM APIs to run your processes directly from your java code.
What You’ll Need
- JBoss Developer Studio w/ JBoss EAP 7.1+ Download here
- JBoss Maven Repositories (Section 1: Steps 4-7)
- Basic Maven & EJB knowledge
Build a KJAR
Step 1: Download and import the simple-process-starter project
- It's a bare java 1.8 maven project
Step 2: Change packaging to kjar
- In your pom.xml, add the jbpm version between the property tags
<jbpm.version>7.11.0.Final-redhat-00004</jbpm.version>
- Add the kie maven plugin in between the build tags
<plugins>
<plugin>
<groupId>org.kie</groupId>
<artifactId>kie-maven-plugin</artifactId>
<version>${jbpm.version}</version>
<extensions>true</extensions>
<dependencies>
<dependency>
<groupId>org.jbpm</groupId>
<artifactId>jbpm-bpmn2</artifactId>
<version>${jbpm.version}</version>
</dependency>
</dependencies>
</plugin>
</plugins>
- Change packaging to kjar (a package structure for workflow files)
<packaging>kjar</packaging>
Step 3: Add a kmodule descriptor
- In src/main/resources/META-INF, create a file called kmodule.xml with the following:
<kmodule xmlns="http://jboss.org/kie/6.0.0/kmodule" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"/>
Step 4: Add a sample process
- Create the folder src/main/resources/com/sample
- Unzip this and copy the process (the bpmn file) into that folder
- If you want to view the process diagram, download the BPM eclipse plugin. I'm using v1.4.3
Step 5: Build & Deploy to Maven Repo
- Right click your project -> Run as -> Maven Build. In the goals section, type:
clean install
Run an Embedded Process
Step 1: Download & import the embedded-process-starter project
- It’s a single page web application. After we’re done, we’ll be able to start a process with one click.
- The pom.xml contains dependencies for running a jBPM process.
- The persistence.xml contains standard objects and queries for jBPM
Step 2: Deploy the KJAR
- Open the StartupBean class. It's an EJB that runs at startup (@Startup)
- Inside this class, declare a DeploymentService EJB
@EJB
DeploymentServiceEJBLocal deploymentService;
- In the init() method, deploy the kjar we just built.
String[] gav = DEPLOYMENT_ID.split(":"); // Splits into group, artifact, and version
DeploymentUnit deploymentUnit = new KModuleDeploymentUnit(gav[0], gav[1], gav[2]);
deploymentService.deploy(deploymentUnit);
- The group, artifact and version specify which artifact we want to deploy
Step 3: Start the process
- In ProcessServlet, declare a ProcessorService EJB
@EJB
private ProcessServiceEJBLocal processService;
- In doPost(), add code to start our process
long processInstanceId = -1;
Map<String, Object> params = new HashMap<String, Object>();
processInstanceId = processService.startProcess(StartupBean.DEPLOYMENT_ID, "com.sample.bpmn.hello", params);
System.out.println("Process instance " + processInstanceId + " has been successfully started.");
Step 4: Build & Deploy to JBoss
- Right click your project -> Run as -> Maven Build.
Goals: clean install
- Deploy using Method 1 here. If you're on EAP 7, be sure to start your server with the full profile:
./standalone.sh --server-config=standalone-full.xml
Step 5: Start a process
- Go to http://localhost:8080/simple-embedded-process/
- Click "Start Process"
- Check the logs. You should see “Hello World!”
Recap
Build a kjar
We created a kjar with our sample process and deployed it to maven
- KJAR (knowledge jar) - a packaged artifact of all our business rules & process files
- kie-maven-plugin - a plugin to compile our processes & create the kjar
- kmodule - a kjar descriptor
Run an Embedded process
1: We declared a DeploymentService to deploy our process.
- The group, artifact and version (GAV) specify the package to deploy.
2: We used ProcessService to start our process.
- com.sample.bpmn.hello is the processId
- When we click “Start Process” on our webpage, it starts this flow. See below
3: Our sample process has a Script Task that prints out “Hello World”

Best Practices for Embedded jBPM
Keep your workflow files (processes, rules) in a separate project from your application code. This will make it easy to change your code & workflows independently.
And, if possible, use JBoss BPM Suite for a centralized repo solution instead of embedding jBPM in your application.
So...
- jBPM APIs allow you to manage complex processes without a dedicated server
- We package processes inside a kjar, deploy it to maven, and run it in our application
- Be sure to keep your process file separate!
Source Code & Useful Links
- Project Source Code (1 & 2)
- BPM User Guide
- Overview of BPM Services API
Happy Coding!
-T.O.