How to Create Business Rule Tasks in jBPM
Business Rule Tasks help us run complex logic within our workflows. Leave those if-statements behind!
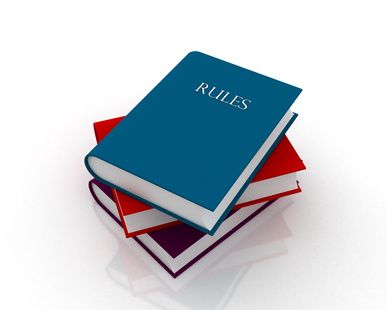
You’re creating business processes for your company using JBPM.
But some of your workflows contain complex logic: regulatory rules, pricing conditions, and approval criteria.
And if you try to write a thousand if-statements, it'll be a nightmare to change any one of them.
So what do you do?
Use Business Rule Tasks
Business rule tasks help us run complex logic within our business process. These tasks make external calls to our rules engine, so our processes remain tidy and easy to change.
Create a Business Process
What You’ll Need
- JBoss Developer Studio w/ JBoss EAP 7.1+ Download here
- JBoss Maven Repositories (Section 1: Steps 4-7)
- Red Hat Process and Rules Development Stack (here)
- Basic Maven & BPM knowledge
We’ll create a fictitious credit card approval process. During the process, we'll check certain credit score and income conditions to see whether a candidate will be approved.
Create a Business Task
Step 1: Download and import simple-rule-process-starter
Step 2: Review the CreditApplication object (src/main/java/CreditApplication)
- This object will hold our credit application. Objects that are inserted into the rule engine are called facts.
Step 3: Review the business process (src/main/resources/com/sample/sample.bpmn)
- Review the Credit Application Process

- Review the creditApplication variable - We’ll use this to store our application
- Right click anywhere in the process → Show property view → Data Items
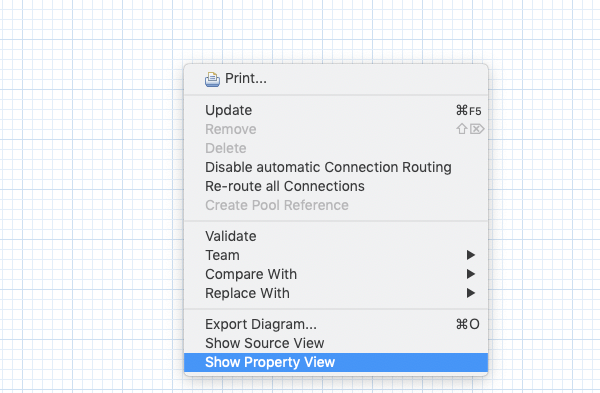
- Script Tasks - Here we setup our credit application and print out the approval status
- Double click the first and last task in the process
Step 4: Add a business rule task
- Mouse over the script task called “CHANGE ME”. Click the lighting rod and select Business Rule Task
- Open the Business Rule Task
- Set the rule-flow-group to credit-approval. A ruleflow-group tells our engine which rules to run
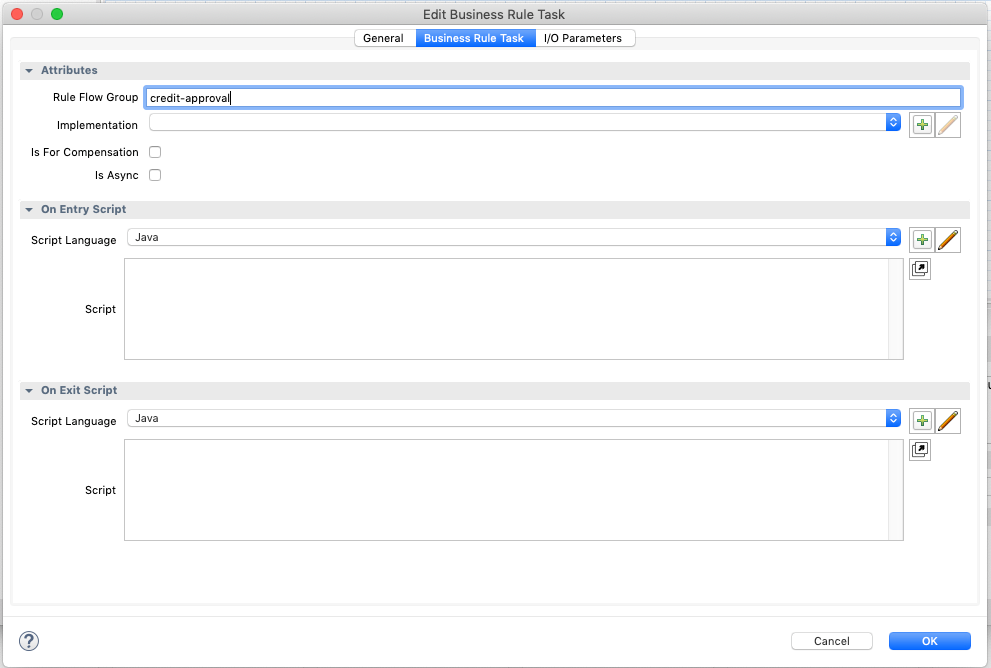
- Under IO params tab, set the input data mapping. We're passing in our creditApplication variable into the rules engine.
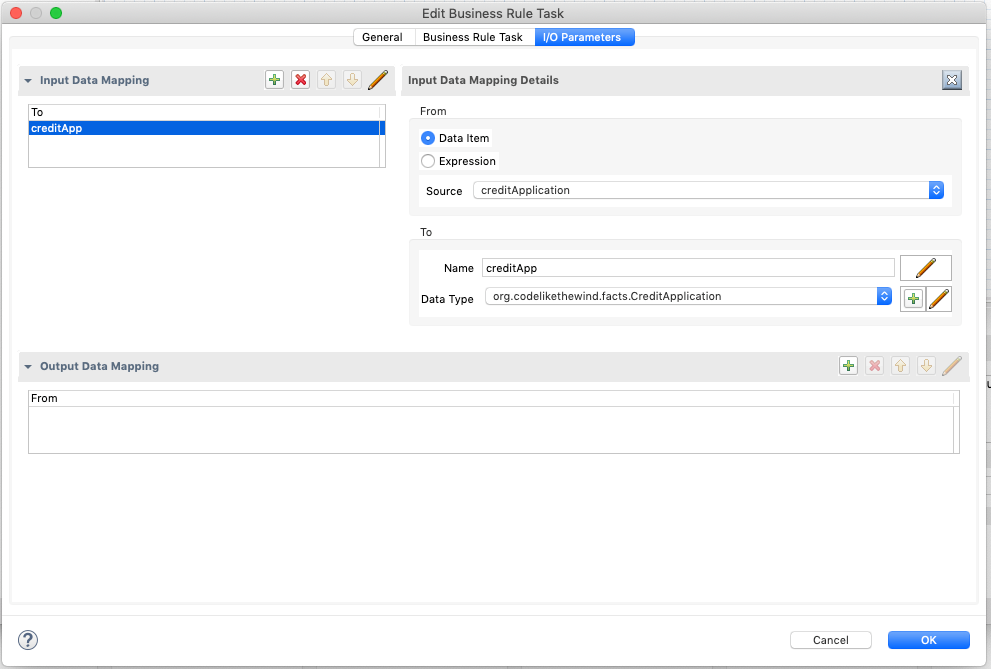
- Set the output data mapping. After the rules fire, this will map the output of the rule execution back to our process variable.
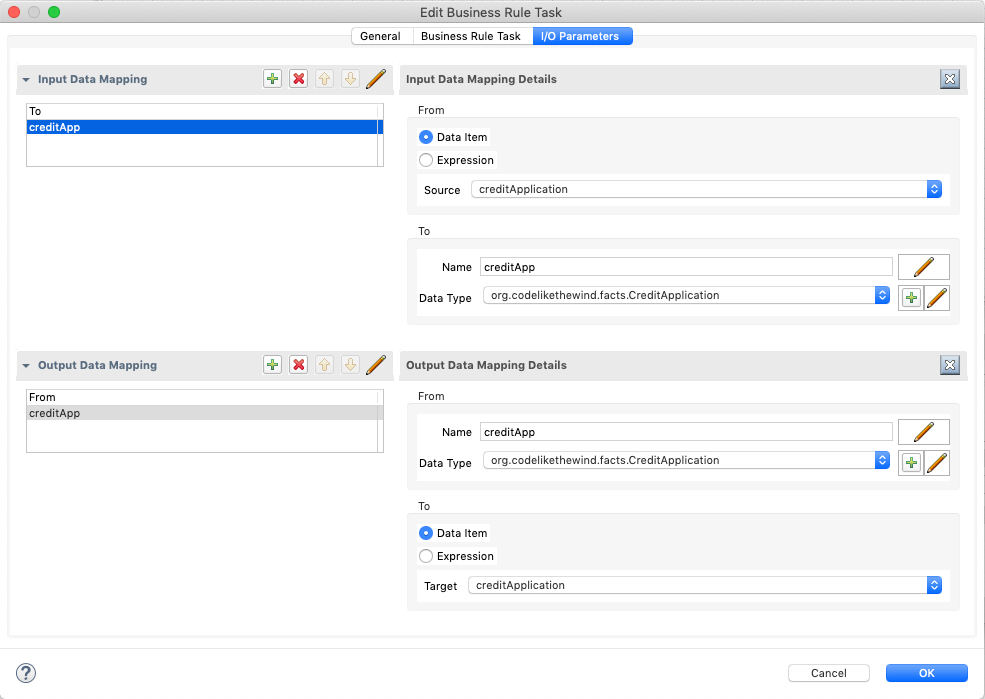
- Under the General tab, change the name to “Credit Approval”
Step 5: Save the file
Create a Business Rule
Step 1: Open the drl file - (src/main/resources/com/sample/rule.drl)
- Drools Rules Language is one language we write rules in
- You can also create your rules using an excel file
Step 2: Write the rule package com.sample
package com.sample
import org.codelikethewind.facts.CreditApplication;
rule "Credit Approval"
ruleflow-group "credit-approval"
no-loop
when
$creditApplication : CreditApplication( creditScore > 500, annualIncome > 50000 )
then
$creditApplication.setApproved(true);
update($creditApplication);
end
Our rule consists of a few things
- rule name → “Credit Approval”
- rule-flow-group → a way to tell engine what rules to run
- no-loop → tell engine to not re-execute this rule
- when → condition of our rule
- then → the action to take
This rule happens to be quite simple. But you can expand this system to execute any number of complex rules.
Step 3: Build the project
mvn clean install
Run the Process
Step 1: Deploy the simple-embedded-rule-process to your server
- Download the project & run mvn clean install
- This simple project runs a deployed process.
- Deploy using Method 1 here. If you’re on EAP 7, be sure to start your server with the full profile:./standalone.sh --server-config=standalone-full.xml
Step 2: Run the Process
- Go to http://localhost:8080/simple-embedded-process/
- Click “Start Process”
Step 3: Verify the rule
- Check the logs. You should see “Credit Application Approved”
Recap
Well Done! Let's review what we just did.
- We’ve created a business process for our fictitious credit application
- We used a business rule task to decide whether we’ll approve a candidate.
- We created a rule that contains logic for our approval. Rules are in “when this, then that” form.
Business rule tasks are a great way to run complex logic in your workflows. They make it easy to change the rules independently of your process.
Source Code & Useful Links
Happy Coding!
-T.O