Q&A: How do we remove version information from EJB JNDI names?
We can use a maven plugin to remove version information from EJB JNDI names.
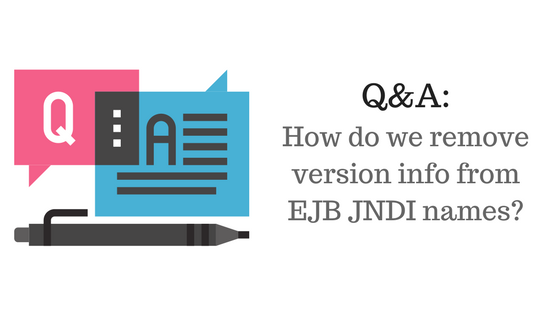
Last week, a client came to me with a dilemma:
"Our build artifacts contain version information to help us know which release we're on. But this information also gets deployed with our EJBs. When we reference a Remote EJB, we have to include the version. For example:
ejb:my-app-ear-1.0.0/my-app-ejb-1.0.0//MyEJB!org.codelikethewind.EJB.RemoteEJB
We don’t want to change our code every time we build a new version, but we still want the version in the filename."
How do we remove version information from EJB JNDI names?
As you remember, the EJB JNDI reference syntax looks like this:
ejb:<app-name>/<module-name>/<distinct-name>/<bean-name>!<fully-qualified-classname-of-the-remote-interface>
We need to remove the version from the application and module name, but leave it on the filename.
Use maven to modify the application and modules names
We can do this with the maven-ear plugin. In the pom.xml of your EAR, you will have something like this (before the </project> tag).
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-ear-plugin</artifactId>
<version>2.10.1</version>
<configuration>
<version>7</version>
<generateApplicationXml>true</generateApplicationXml>
<!-- remove the version in the application name -->
<applicationName>{project.artifactId}</applicationName>
<modules>
<!-- remove the version in the module name -->
<ejbModule>
<groupId>${project.groupId}</groupId>
<artifactId>MyEJB</artifactId>
</ejbModule>
</modules>
</configuration>
</plugin>
</plugins>
</build>
How to Set it Up
Step 1: Add the maven-ear plugin
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-ear-plugin</artifactId>
<version>2.10.1</version>
</plugin>
Step 2: Change the application name
- Generate an application.xml for your java version
<version>7</version>
<generateApplicationXml>true</generateApplicationXml>
- Remove the version from the application-name
<applicationName>{project.artifactId}</applicationName>
- application.xml- a deployment descriptor that specifies metadata for your app
Step 3: Change the module name
- Add an ejbModule tag with a groupId and artifactId (for each of your EJBs)
<modules>
<ejbModule>
<groupId>${project.groupId}</groupId
<artifactId>MyEJB</artifactId>
</ejbModule>
</modules>
- The artifactId should have no version info
Step 4: Build the project
- Right click your project -> Run as -> Maven Build
Goals: clean install
Step 5: Deploy the project onto your server
- You should see the JNDI reference strings without any version information
I hope this can save you some time.
Happy Coding,
-T.O.